Initial Render slightly slower than Vue 2 in Vue-CLI base app.
Environment
Chrome 88.0.4324.96(正式版本) (x86_64) iMac (Retina 5K, 27-inch, 2017) Mac OS Mojave Vue CLI 4.5.9 Node.js 12.13.1
Version
3.0.0
Reproduction link
https://github.com/techbirds/learn-vue
Code Preivew
<template>
<div id="app">
<!-- <img alt="Vue logo" src="./assets/logo.png"> -->
<HelloWorld msg="Welcome to Your Vue.js App" v-for="n in 3000" :key="n"/>
</div>
</template>
<script>
import HelloWorld from './components/HelloWorld.vue'
export default {
name: 'App',
components: {
HelloWorld
}
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
Steps to reproduce
1、use vue cli to create v3 app and v2 app
2、render HelloWorlds component 3000 times
3、watch performance with chrome devtools。
v2 perf:
v3 perf:
project link:
https://github.com/techbirds/learn-vue/tree/master/vue2-demo https://github.com/techbirds/learn-vue/tree/master/vue3-demo
What is expected?
v3 faster than v2
What is actually happening?
The speed is about the same, even slower than v2
Tested with a project using vite and vue 3 and then the same components using vue-cli with vue 2, after bundling in prod mode.
Vue 3
Vue 2
You can find other benchmarks at https://github.com/krausest/js-framework-benchmark
Just to add: it doesn't make too much sense to compare performance in development mode (npm run serve
). Vue 3 could very well be a tad slower as Vue 2, but that would not matter unless it was so bad that it slowed down your development work, which it isn't.
What's important is the production build.
1、on the production build with npm run build
2、run as http-server
v2 perf:
v3 perf:
v3 performance slower than v2.
please tell me why ? Is the test posture wrong?
Tested with a project using vite and vue 3 and then the same components using vue-cli with vue 2, after bundling in prod mode.
Vue 3
Vue 2
You can find other benchmarks at https://github.com/krausest/js-framework-benchmark
Can you provide code samples and test procedures?
I used the code generated by yarn create @vitejs/app
and migrated it to options api with Vue CLI and Vue 2 to compare. As @LinusBorg pointed out in discussions, it could be related to differences between the bundling (webpack vs rollup). Maybe there is something to improve here but not sure where
Test:
without webpack bundle. run with only vue framework.
v2
v3
Phenomenon:
- Based on the production build of vue cli, the running performance of v2 and v3 is very close
- Based on pure HTML online template compilation and construction:
- The running performance of v3 is much better on prod than v2 prod
- The development version of v3 is very close to the prod version of v2
- There is a certain gap in performance between the development version of v2 and the prod version of v2, but it is obviously not as big as the gap between v3
Problem:
- Why is the production build performance based on vue cli so poor?
- What is the difference between the production and development versions of v3? Why is the performance poor so bad?
Code Sample:
https://github.com/techbirds/learn-vue/blob/master/html/index.v2.html https://github.com/techbirds/learn-vue/blob/master/html/index.v3.html
Noteworthy is that this is only looking at the initial render. Efficiency of updates is another, arguably maybe more important thing for performance.
Also, on the upside, this shows how mouch more efficient Vue 3 is memory-wise. In these measurements, memory consumption is cut in half compared to Vue 2.
Investigated a bit and turns out the perf bottleneck is due to static content insertion. The component is largely static so it compiles into a single static node with an HTML string. When mounting such a node, we are currently setting it as innerHTML
of a temp container and then move the created nodes into the mount target. Turns out this is actually much slower than just normal VDOM mounting. We will need to adjust either the static insertion logic or the stringification strategy. It is still important for SSR hydration performance.
Vue 2
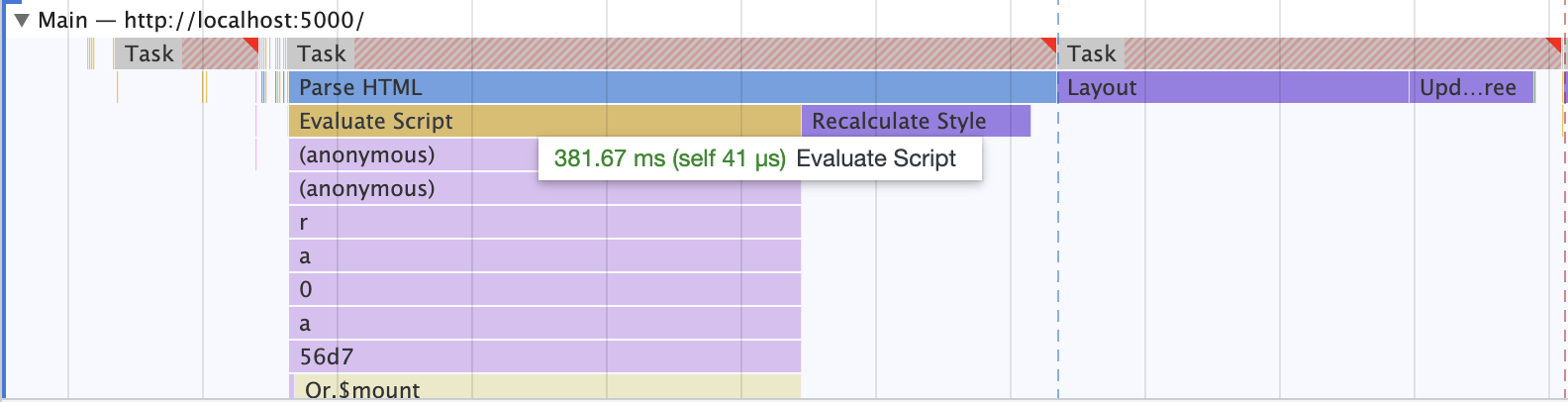
Vue 3 before fix
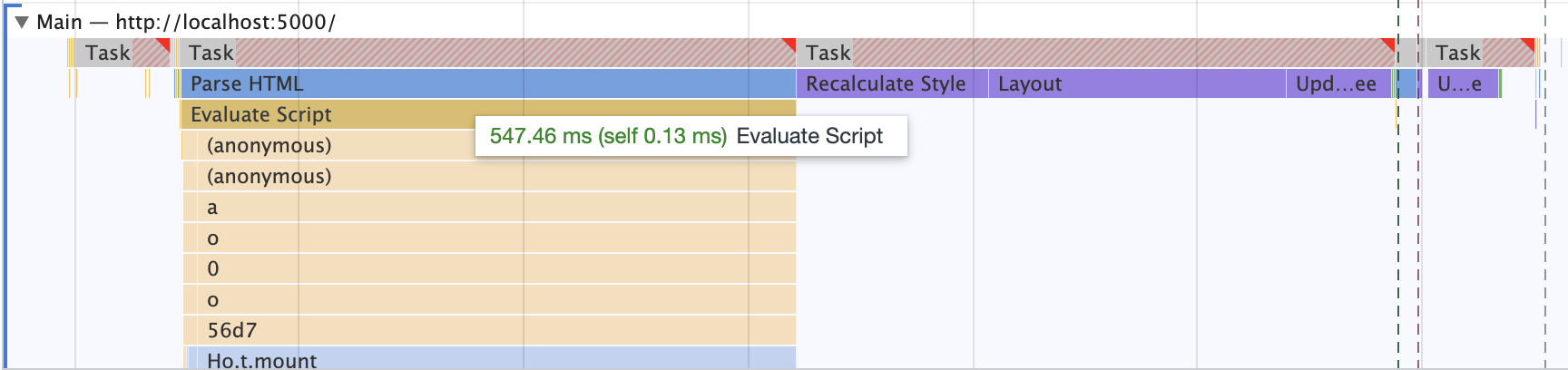
Vue 3 after fix
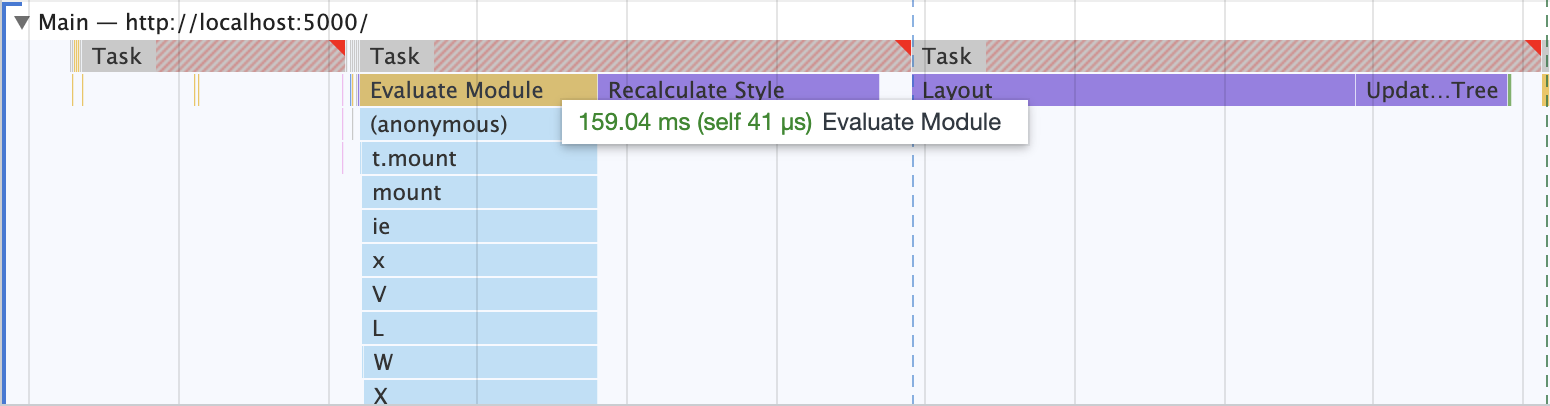